Overview
In web development, we often need to store and manage various data on the client side. In particular, when communicating with a server, we can store and use credentials and session information in the browser, and the browser provides several techniques for doing so. In this post, I will introduce the different ways to store data in the browser and their characteristics.
What is a cookie
A term you’ve probably heard a lot, both good and bad, cookies are data that is stored in your browser when you visit a website. Cookies stored by a particular site can be sent back to the server and are used by the website to remember your web browser.
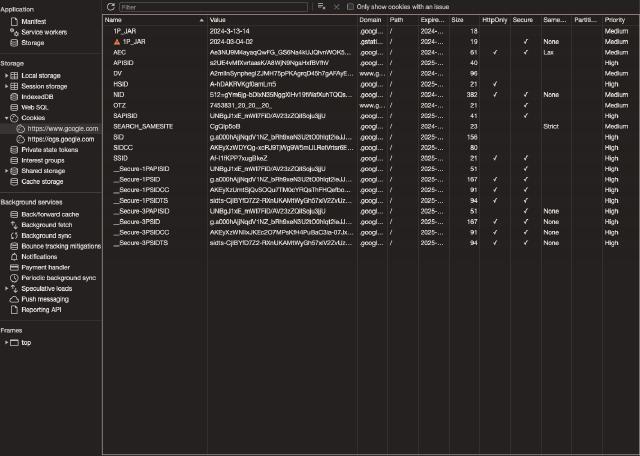
How it works
- a user visits a website.
- the website server asks the user’s browser to store a cookie.
- The browser saves this cookie on the user’s device.
- When the user visits the same website again, the browser sends this cookie back to the server, which uses the cookie information to identify the user.
Properties
Cookies can have the following attributes
Value
: This is the part that stores the actual data.Expires/Max-Age
: Determines how long the cookie will live. If not set, it will be a session cookie and will be deleted when you close the browser.Domain
: Specifies the domain from which the cookie can be sent. By default, it is set to the domain of the site that generated the cookie.Path
: Specifies the path where the cookie can be sent.Secure
: If this property is set, cookies are sent only over the HTTPS protocol.HttpOnly
: Prohibits cookies from being accessed via JavaScript. This helps prevent XSS attacks via cookies.
Server Example (Golang)
Using the golang echo package, I wrote a simple code to generate a cookie and send it to the client.
1package main
2
3import (
4 "github.com/labstack/echo/v4"
5 "net/http"
6 "time"
7)
8
9func main() {
10 e := echo.New()
11
12 e.POST("/login", func(c echo.Context) error {
13 // Generate the JWT after the user authentication logic (here we put our own token as an example)
14 token := "your.jwt.token.here"
15
16 cookie := new(http.Cookie)
17 cookie.Name = "jwt"
18 cookie.Value = token
19 cookie.Expires = time.Now().Add(24 * time.Hour)
20 cookie.HttpOnly = true // prevent client scripts from accessing the cookie
21
22 c.SetCookie(cookie)
23
24 return c.String(http.StatusOK, "Login Successful and JWT stored in cookie")
25 })
26
27 e.Logger.Fatal(e.Start(":8080"))
28}
You can prevent scripts on the client from accessing the cookie via a field called HttpOnly.
What is Web Storage
This is a data storage mechanism provided by the browser that is stored in the browser similar to cookies, but unlike cookies, it is not automatically sent to the server. There is local storage and session storage, which can be stored in key/value form, and IndexedDB, which can store more complex forms of data, but we’ll cover that later.
Local storage
Allows you to store data persistently in the user’s browser, and the data persists between browser sessions, meaning it doesn’t disappear when the user closes and reopens the browser.
**Features
- Can store large amounts of data (typically 5MB to 10MB).
- Not automatically sent to the server.
- Stored separately by domain.
- Not shared between HTTP and HTTPS content.
Local storage examples
How to use local storage to store and read back a user’s language preference settings.
1// Store data in local storage
2localStorage.setItem('preferredLanguage', 'en');
3
4// Read data from local storage
5const preferredLanguage = localStorage.getItem('preferredLanguage');
6console.log(preferredLanguage); // print 'en'
7
8// Delete data from local storage
9localStorage.removeItem('preferredLanguage');
10
11// Delete all data from local storage
12localStorage.clear();
Session storage
Session storage stores data until the user closes the browser tab, meaning that data is only retained as long as the tab or window is open.
Features
- Data is destroyed when the tab or window is closed.
- Can store large amounts of data, similar to local storage.
- Not automatically sent to the server.
- Managed uniquely by each tab or window.
Session storage examples
How to use session storage to store and read back a user’s temporary shopping cart ID.
1// Store data in session storage
2sessionStorage.setItem('cartId', '12345');
3
4// Read data from session storage
5const cartId = sessionStorage.getItem('cartId');
6console.log(cartId); // print '12345'
7
8// Delete data from session storage
9sessionStorage.removeItem('cartId');
10
11// Delete all data from session storage
12sessionStorage.clear();
Cookies vs Web Storage
It’s hard to say which is “better”: web storage or cookies. Depending on your use case, you’ll need to decide which is better for you. For example, if you want to use large amounts of data only on the client side, web storage might be better for you. On the other hand, if you need to maintain state with the server or have security-critical data, you might be better off using cookies.
As explained above, the HttpOnly option is present in cookies, whereas web storage does not have that feature, which can make it vulnerable to cross-site scripting (XSS) attacks.
Summary
We’ve seen how there are different ways to store data in the browser and how you can access and store it through examples. As mentioned above, there are similarities, but it’s important to find the right method for your purposes.